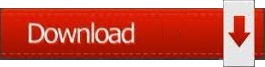
Enqueue FunctionĮnqueue function adds an element to the end of the queue. Steps for implementing queue using linked list: 1. Implementing a queue using a linked list allows us to grow the queue as per the requirements, i.e., memory can be allocated dynamically.Ī queue implemented using a linked list will not change its behavior and will continue to work according to the FIFO principle. Implementation of queue using linked list in C If the queue is empty, then the new node added will be both front and rear, and the next pointer of front and rear will point to NULL. Now, two conditions arise, i.e., either the queue is empty, or the queue contains at least one element. ptr = (struct node *) malloc (sizeof(struct node)) Algorithm to perform Insertion on a linked queue:Ĭreate a new node pointer. The new element which is added becomes the last element of the queue. Insert operation or insertion on a linked queue adds an element to the end of the queue. The two main operations performed on the linked queue are: If front and rear both points to NULL, it signifies that the queue is empty. Insertion is performed at the rear end, whereas deletion is performed at the front end of the queue. The front pointer stores the address of the first element of the queue, and the rear pointer stores the address of the last element of the queue. The data field of each node contains the assigned value, and the next points to the node containing the next item in the queue.Ī linked queue consists of two pointers, i.e., the front pointer and the rear pointer. A good example of a queue is a queue of customers purchasing a train ticket, where the customer who comes first will be served first.Įach node of a linked queue consists of two fields: data and next(storing address of next node). What is Queue?Ī queue is a linear data structure that follows the First In, First Out (FIFO) principle, which means that the element which is inserted first in the queue will be the first one to be removed from the queue. The benefit of implementing a queue using a linked list over arrays is that it allows to grow the queue as per the requirements, i.e., memory can be allocated dynamically. It can be implemented using an array and linked list. Queue supports operations like enqueue and dequeue. Introduction to Implementation of Queue using Linked ListĪ queue is a linear data structure that follows the First in, First out principle(FIFO). We learn about various operations performed on queue like enqueue and dequeue.
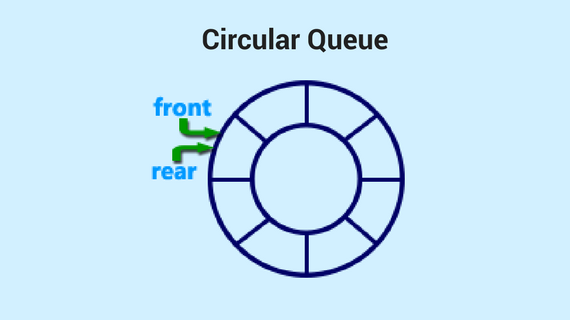
This article defines the implementation of a queue using linked list in C language.The queue rule says that insertion takes place at one end and deletion takes place at the other end, i.e., First In, First Out(FIFO).īefore reading this article, understand the following C Programming topics:
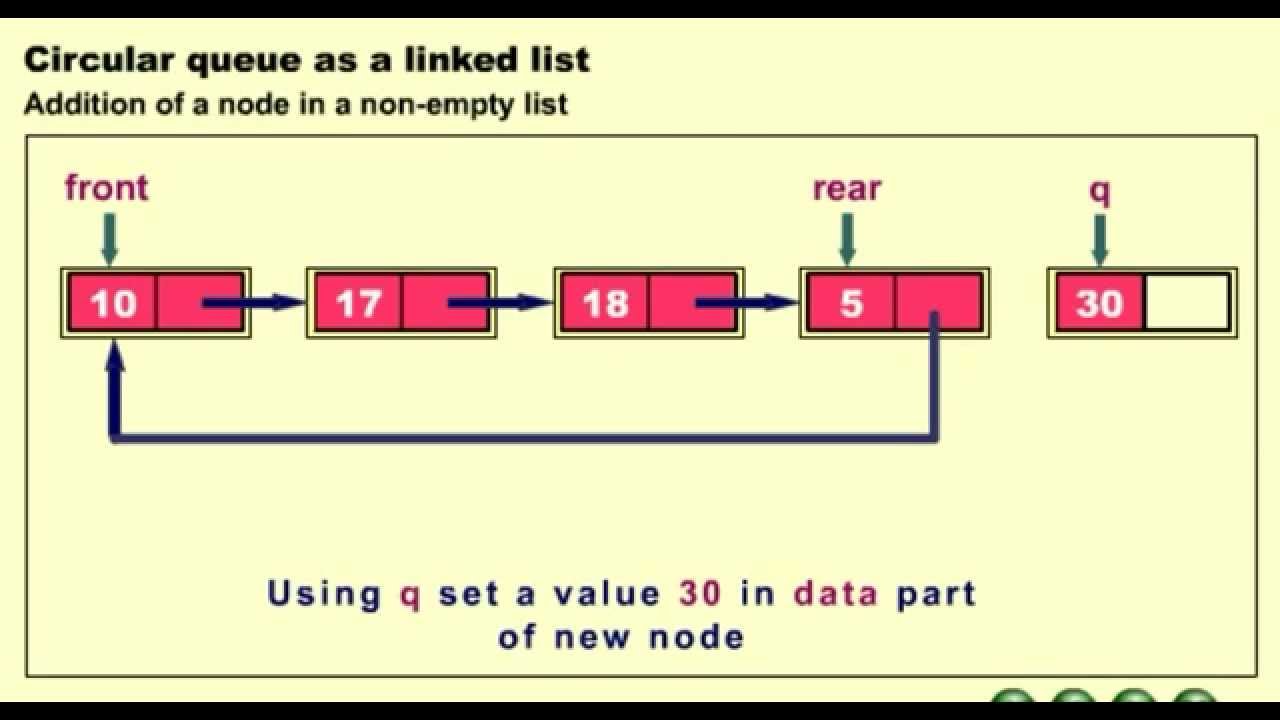
Using a linked list means that we are going to store the information in the form of nodes following the rules of the queue.

Here is java program to implement queue using linked list.In this article, we will learn about the implementation of queue data structure using a Linked List in C language. Queue can be implemented by stack, array and linked list. Queue: Queue is a data structure, that uses First in First out(FIFO) principle. Here is a java program to implement stack using linked list. Stack: What is stack? Stack is a linear data structure which implements data on last in first out criteria. Two popular applications of linked list are stack and queue. Each node has a value and a link to next node. The implementation of a linked list is pretty simple in Java.
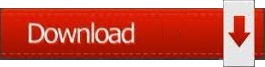